Featured!
Using SureCloud’s automated evidence collection to streamline ISO 27001 compliance
Streamline your ISO 27001 compliance with SureCloud's automated evidence collection, reducing manual work and enhancing audit efficiency.
.jpg)
Featured!
Essential GRC Glossary: 30+ Key Governance, Risk & Compliance Terms
Explore 30+ essential GRC terms every compliance and risk leader should know. Learn how SureCloud helps simplify governance, risk, and compliance.
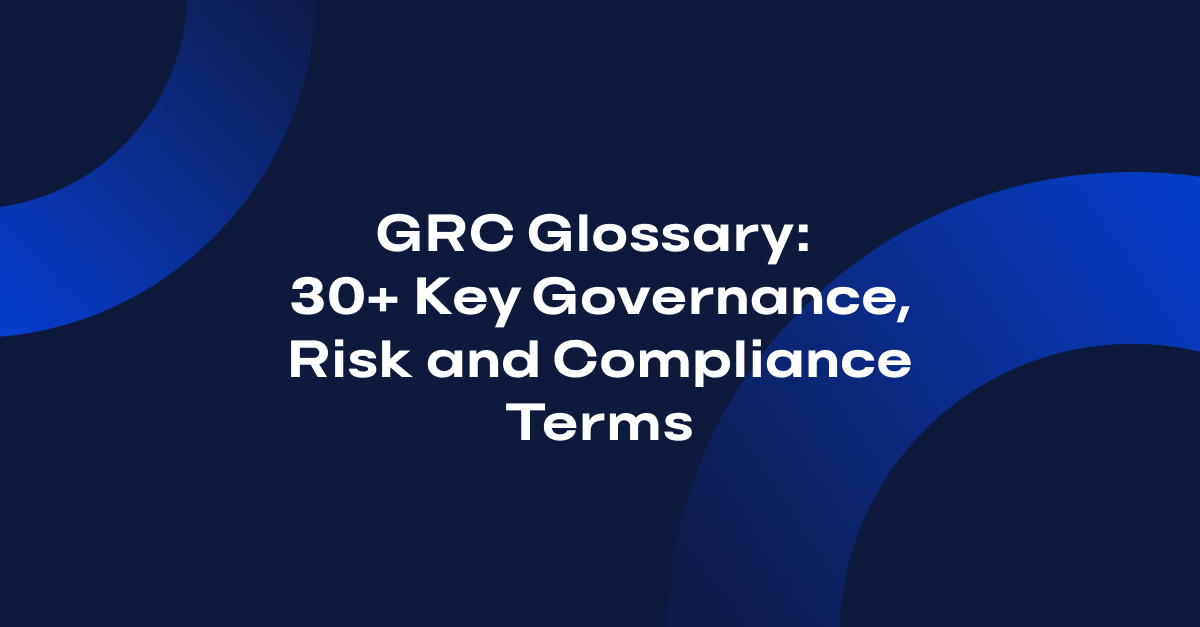
Featured!
2025 Risk, Compliance, Cybersecurity & GRC Events - SureCloud
Join SureCloud at leading 2025 cybersecurity, risk, and GRC events. Book demos, hear expert talks, and explore solutions for your compliance challenges.
.png)
Featured!
Specsavers frames the future of Security GRC with SureCloud
Specsavers partners with SureCloud to enhance security GRC, streamline processes and improve efficiency across its global operations.
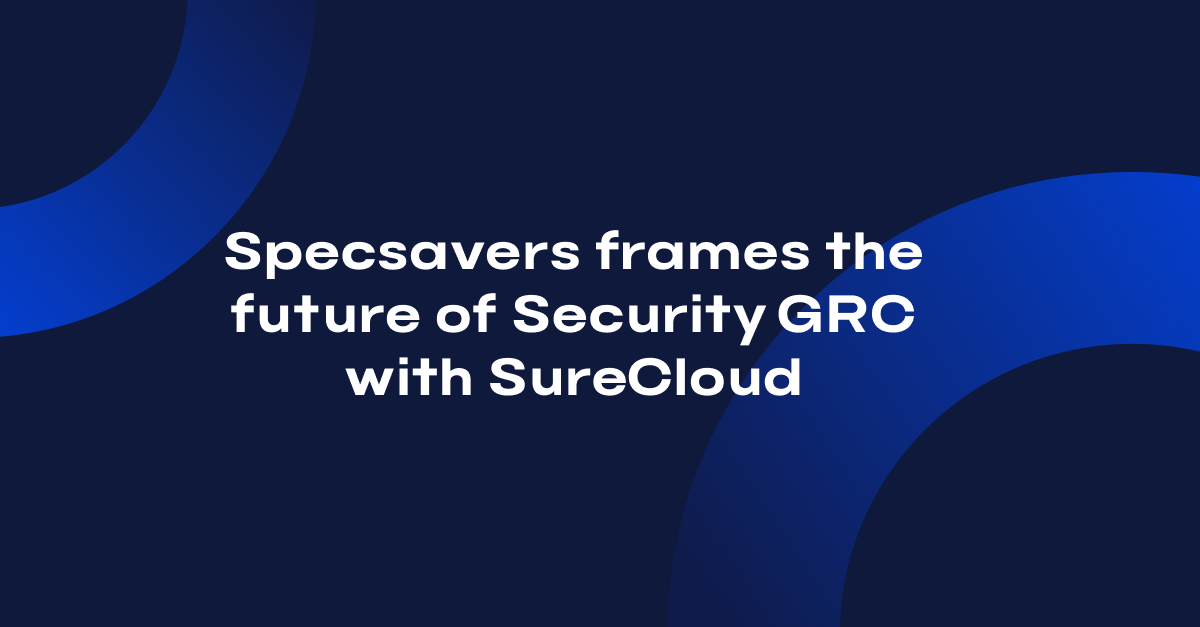
Stay in the know with SureCloud
Want to keep your fingers on the pulse of the information security world? Subscribe to the SureCloud newsletter and get the latest news, resources and insights – straight to your inbox.